Xamarin Splash Screen
- Vlad Zakharo
- Apr 13, 2022
- 3 min read
Updated: Apr 14, 2022
A splash screen, also known as a launch screen, is the first screen that a user sees when opening your app, and it stays visible while the app is loading. I propose to make the loading process more interesting, dynamic and animated.
Of course, if you are developing a large application, then sooner or later the question arises about creating a loading screen.
So let's look at how to work with the splash screen in the Xamarin application for Android and iOS.
First, you need to know that the loading screen in Xamarin must be implemented on each platform, because common implementation is impossible until Xamarin.Forms is not initialized.
Static image
Microsoft has given very detailed instructions on how to create a splash screen for Android and iOS. You will get a similar result:
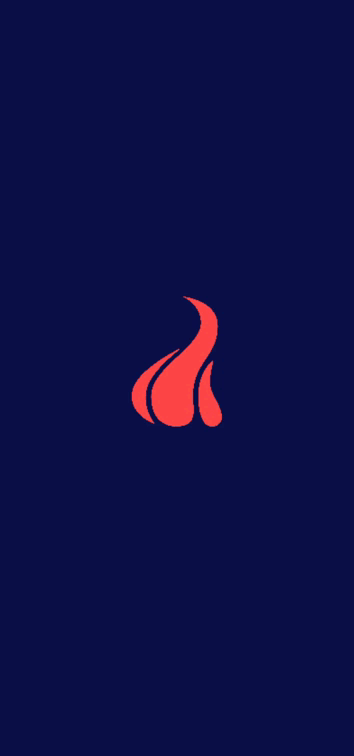
But I want to focus on some things.
Android
I would advise you to spend more time on google documentation, like this to create a drawable for the Splash Screen and article about Styles and Themes.
iOS Some Windows developers are having trouble opening a storyboard file and editing it directly from within Windows. If you are one of them, your solution is to create LaunchScreen.storyboard in Xcode from under your Mac and copy the resulting file to your windows project.
Animated splash screen
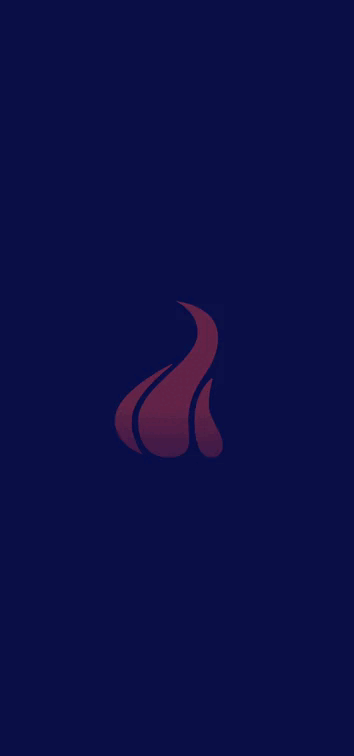
I use Lottie to add an Animated splash screen. Firstly, you need to add Lottie packages to your projects:

If you want to use pre-made animations, you can choose them from lottiefiles.com or create your own using Bodymovin After Effects extension to export Lottie animations. Animations are exported by default as .json. You can render animations in the browser on svg, canvas and html. It supports a subset of After Effects features. Animations can also be played natively on iOS and Android using Lottie.
The resulting json file must be added to native projects:
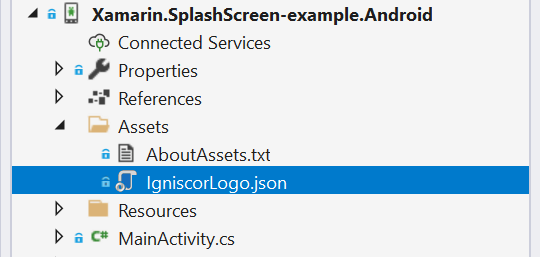
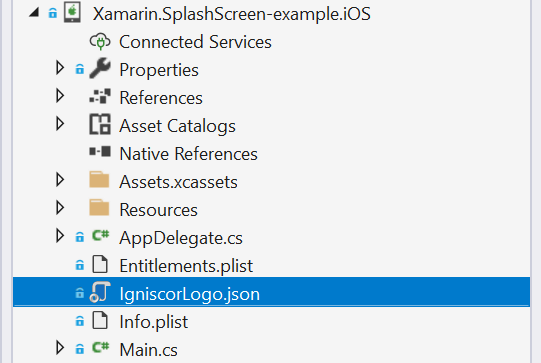
Android
First, you need to create an xml file with LottieAnimationView, you can experiment with its attributes. Of course, it must contain a link to the file that you want to use as an animation with a json extension:
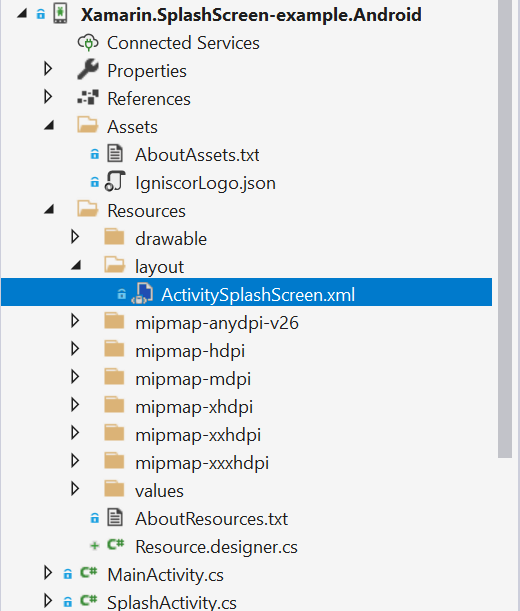
app:lottie_fileName="IgniscorLogo.json"
ActivitySplashScreen.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.airbnb.lottie.LottieAnimationView
android:id="@+id/animation_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:lottie_fileName="IgniscorLogo.json"
app:lottie_autoPlay="true"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="10.0dp"
android:layout_marginRight="10.0dp"
android:layout_marginTop="10.0dp"
android:layout_marginLeft="10.0dp" />
</RelativeLayout>
Go to SplashActivity.cs
In the OnCreate method, you need to set LottieAnimationView and add listeners.
SetContentView(Resource.Layout.ActivitySplashScreen);
var animationView = FindViewById<LottieAnimationView>(Resource.Id.animation_view);
animationView.AddAnimatorListener(this);
You also need to implement the Animator.IAnimatorListener interface:
public void OnAnimationCancel(Animator animation)
{
}
public void OnAnimationEnd(Animator animation)
{
StartActivity(new Intent(Application.Context,
typeof(MainActivity)));
}
public void OnAnimationRepeat(Animator animation)
{
}
public void OnAnimationStart(Animator animation)
{
}
After the animation completes, MainActivity starts.
iOS
In the Info.plist change "Launch Screen" state to "(not set)".
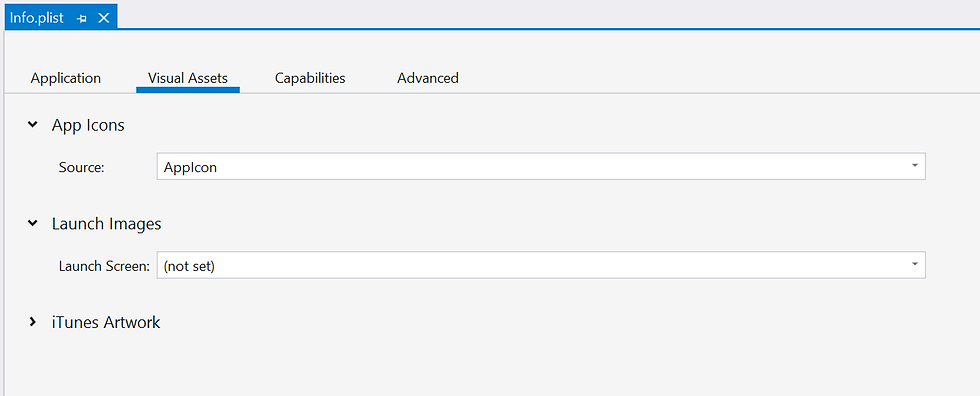
Create SplashViewController class with next code:
public class SplashViewController : UIViewController
{
public SplashViewController() : base() { }
public override void ViewDidLoad()
{
base.ViewDidLoad();
var viewAnimation =
LOTAnimationView.AnimationNamed("IgniscorLogo");
viewAnimation.ContentMode = UIViewContentMode.Center;
View.AddSubview(viewAnimation);
View.BackgroundColor = UIColor.FromRGB(12, 18, 72);
viewAnimation.Center = View.Center;
viewAnimation.PlayWithCompletion((finished) =>
{
UIApplication.SharedApplication.Delegate.FinishedLaunching
(UIApplication.SharedApplication, new
Foundation.NSDictionary());
});
}
}
Let's change the contents of the FinishedLaunching method in the AppDelegate class:
public override bool FinishedLaunching(UIApplication app, NSDictionary options)
{
if (Window == null)
{
Window = new UIWindow(frame:
UIScreen.MainScreen.Bounds);
var initialViewController = new SplashViewController();
Window.RootViewController = initialViewController;
Window.MakeKeyAndVisible();
return true;
}
else
{
global::Xamarin.Forms.Forms.Init();
AnimationViewRenderer animationView = new
AnimationViewRenderer();
animationView.Init();
LoadApplication(new App());
return base.FinishedLaunching(app, options);
}
}
When FinishedLaunching is called for the first time, the animation will start, and when it finishes, Xamarin.Forms will be initialized and the LoadApplication method will be called.
If you want to see all the examples in this article, check out the repository. Thank you for your attention.
Comments